What is Dioxus, How to Build?
Dec 11, 2024Build for web, desktop, and mobile, and more with a single codebase. Zero-config setup, integrated hot-reloading, and signals-based state management. Add backend functionality with Server Functions and bundle with our CLI.
Dioxus: A Fullstack Cross-Platform App Framework for Rust
Dioxus is a fullstack and cross-platform framework for building web, desktop, mobile, and server apps using Rust. It offers a React-like development experience with features like components, props, and hooks, but with Rust's performance and safety. Key features include ergonomic state management, type-safe routing, an integrated bundler, and instant hot-reloading. Dioxus supports various platforms, including web (with SSR and hydration), desktop (macOS, Linux, Windows), and mobile (Android, iOS).
Building with Dioxus: A Step-by-Step Guide
The process of building with Dioxus involves several key steps:
1. Prerequisites:
- An Editor: Dioxus works well with editors supporting the Rust-Analyzer LSP plugin for enhanced code assistance.
- Rust: Install the Rust compiler from https://rust-lang.org. It's highly recommended to work through the official Rust book (https://doc.rust-lang.org/book/ch01-00-getting-started.html) for a solid foundation.
2. Installation:
Install the Dioxus CLI (dx
) using:
cargo install dioxus-cli
If you encounter OpenSSL errors, ensure the necessary dependencies are installed (refer to https://docs.rs/openssl/latest/openssl/#automatic for details).
3. Creating a New Project:
Use the CLI to create a new project:
dx new my-app
cd my-app
You'll be prompted to select a platform (Web, Desktop, Mobile, etc.), a styling library (e.g., vanilla CSS), and whether to enable routing.
4. Running Your App:
Start the development server:
dx serve
For LiveView templates, use dx serve --desktop
. For web targets, the application will be served at a specified address.
5. Core Concepts:
- Components: Reusable UI elements built using functions returning
Element
. The#[component]
attribute simplifies component creation and prop management. rsx!
Macro: A JSX-like macro for creating elements with attributes, listeners, and children. Attributes and listeners are listed before children.- Hooks: Reusable logic units for managing state and side effects within components. They start with
use_
(e.g.,use_signal
). Adhere to the "rules of hooks" for correct usage.
6. Example: A Simple Counter
A basic Dioxus counter app demonstrates core concepts:
use dioxus::prelude::*;
#[component]
fn App() -> Element {
let mut count = use_signal(|| 0);
rsx! {
div { "Count: {count}" }
button { onclick: move |_| count += 1, "Increment" }
button { onclick: move |_| count -= 1, "Decrement" }
}
}
7. Further Learning:
Explore the Dioxus documentation (https://dioxuslabs.com/learn/0.6/) for tutorials, guides, and advanced topics like server-side rendering, concurrent rendering, and platform-specific details. The Dioxus website also serves as a testbed for new features.
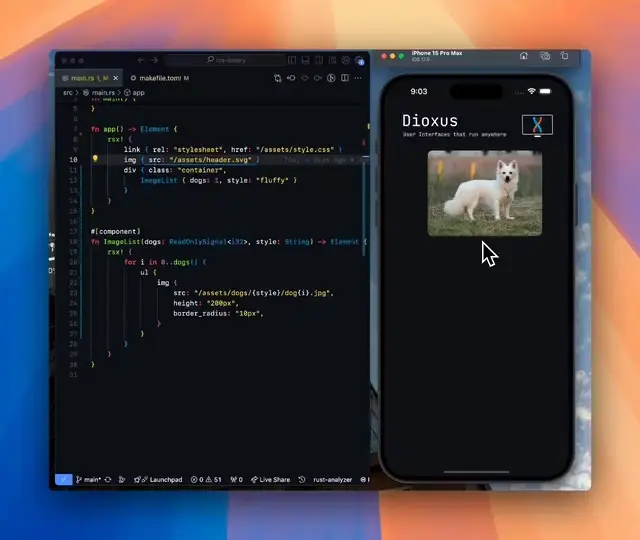




Exploring the Landscape of AI Web Browsing Frameworks
Published Jan 24, 2025
Explore the landscape of AI web browsing frameworks, from browser-integrated assistants to dedicated automation platforms. Learn how these tools are transforming the web experience with intelligent content extraction, task automation, and user-friendly interfaces....
OpenAI Operator: A New Era of AI Agentic Task Automation
Published Jan 23, 2025
Explore OpenAI Operator, a groundbreaking AI agent automating tasks by interacting with computer interfaces. Discover its capabilities, limitations, and impact on the future of AI....
React OpenGraph Image Generation: Techniques and Best Practices
Published Jan 15, 2025
Learn how to generate dynamic Open Graph (OG) images using React for improved social media engagement. Explore techniques like browser automation, server-side rendering, and serverless functions....